Hi everyone, inside this article we will see about C++ Recursion.
Recursion is a technique in computer programming where a function calls itself in order to solve a problem. It’s a powerful technique that can be used to solve problems that can be broken down into smaller sub problems.
In C++, recursion is implemented by having a function call itself, typically with a different set of inputs, until it reaches a base case. The base case is the point at which the recursion stops and the function returns a result.
The syntax of C++ recursion involves defining a function that calls itself, typically with a different set of inputs, until it reaches a base case. The base case is the point at which the recursion stops and the function returns a result.
Here is the basic syntax for implementing recursion in C++:
int function_name(int n) {
// Base case
if (n == base_value) {
return result;
}
// Recursive case
else {
return function_name(n - 1);
}
}
In this example, the function function_name
takes an argument n
and checks if it is equal to the base value base_value
. If n
is equal to base_value
, the function returns the result. If n
is not equal to base_value
, the function calls itself with a different value of n
(in this case, n - 1
), and the process repeats until the base case is reached.
Example
Here’s an example of a recursive function in C++ that calculates the factorial of a given number:
#include <iostream> using namespace std; int factorial(int n) { if (n == 0) { return 1; } else { return n * factorial(n - 1); } } int main() { int num = 5; cout << "The factorial of " << num << " is " << factorial(num) << endl; return 0; }
Output
The factorial of 5 is 120
Pictorial view of this above program.
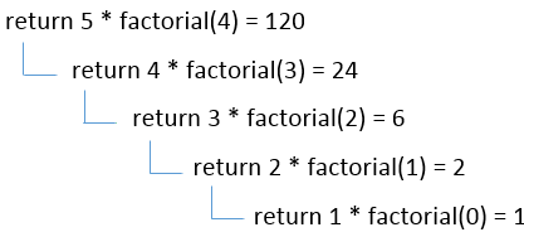
We hope this article helped you to understand about C++ Recursion in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.