Hi everyone, inside this article we will see about C++ if-else Statement.
The if-else
statement is a control structure in C++ that allows you to conditionally execute a block of code based on the truth value of a specified condition. If the condition is true, the code in the if
block is executed. If the condition is false, the code in the else
block is executed.
Here’s the syntax of an if-else
statement in C++:
if (condition) {
// code to be executed if condition is true
} else {
// code to be executed if condition is false
}
Here’s an example of using an if-else
statement in C++:
#include <iostream> using namespace std; int main() { int num = 10; if (num > 0) { cout << num << " is positive." << endl; } else { cout << num << " is negative or zero." << endl; } return 0; }
Output
10 is positive.
Types of if Statements in C++
There are several types of if
statements in C++, including:
Simple if
statement
It Executes a block of code if a specified condition is true.
Syntax
if (condition) {
// code to be executed if condition is true
}
Note: The code inside { }
is the body of the if
statement.
A bit more analysis by pictorial view:
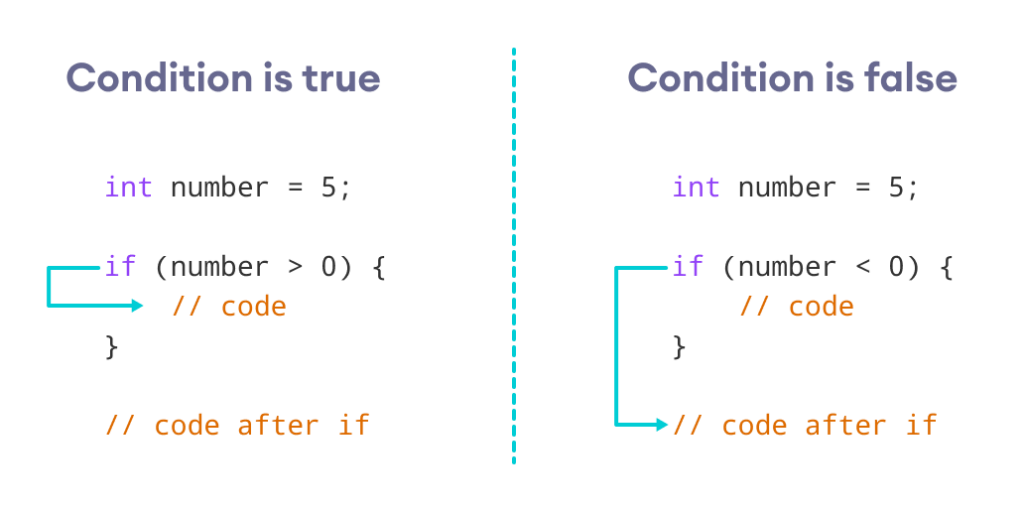
Program
// Program to print positive number entered by the user // If the user enters a negative number, it is skipped #include <iostream> using namespace std; int main() { int number; cout << "Enter an integer: "; cin >> number; // checks if the number is positive if (number > 0) { cout << "You entered a positive integer: " << number << endl; } cout << "This statement is always executed."; return 0; }
Output
Enter an integer: 5
You entered a positive number: 5
This statement is always executed.
When the user enters 5
, the condition number > 0
is evaluated to true
and the statement inside the body of if
is executed.
if-else
statement
An if-else
statement allows you to specify two different blocks of code to be executed based on the result of a condition. If the condition is true, the first block of code is executed, otherwise, the second block of code is executed.
Syntax
if (expression) {
// code to be executed if expression is true
} else {
// code to be executed if expression is false
}
A bit more analysis by pictorial view:
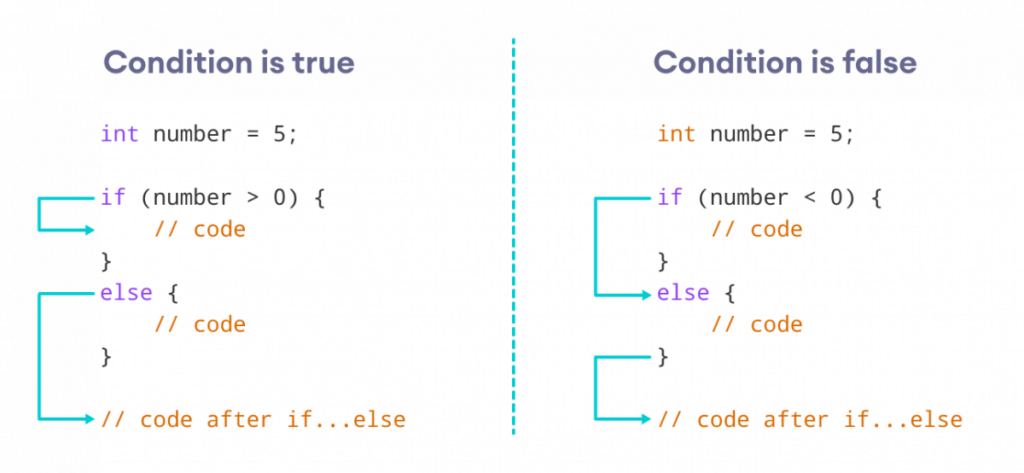
Program
// Program to check whether an integer is positive or negative // This program considers 0 as a positive number #include <iostream> using namespace std; int main() { int number; cout << "Enter an integer: "; cin >> number; if (number >= 0) { cout << "You entered a positive integer: " << number << endl; } else { cout << "You entered a negative integer: " << number << endl; } cout << "This line is always printed."; return 0; }
Output
Enter an integer: -4
You entered a negative integer: -4
This line is always printed.
Here, we enter -4. So, the condition is false
. Hence, the statement inside the body of else
is executed.
if-elseif-else
ladder statement
An if-else if-else
statement allows you to specify multiple conditions and execute different blocks of code based on the result of each condition. This statement is useful when you have multiple conditions that need to be checked and only one block of code needs to be executed.
Syntax
if (expression1) {
// code to be executed if expression1 is true
} else if (expression2) {
// code to be executed if expression1 is false and expression2 is true
} else {
// code to be executed if both expression1 and expression2 are false
}
A bit more analysis by pictorial view:
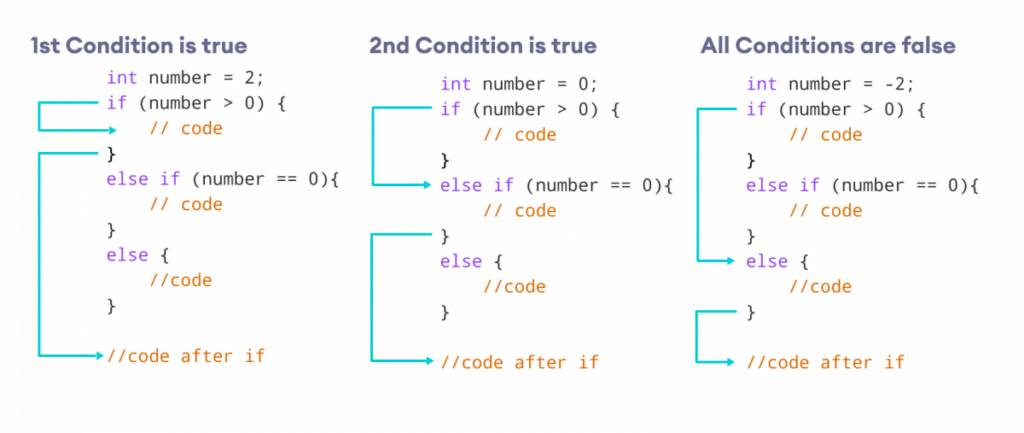
Program
// Program to check whether an integer is positive, negative or zero #include <iostream> using namespace std; int main() { int number; cout << "Enter an integer: "; cin >> number; if (number > 0) { cout << "You entered a positive integer: " << number << endl; } else if (number < 0) { cout << "You entered a negative integer: " << number << endl; } else { cout << "You entered 0." << endl; } cout << "This line is always printed."; return 0; }
Output
Enter an integer: -2
You entered a negative integer: -2
This line is always printed.
In this program, we take a number from the user. We then use the if...else if...else
ladder to check whether the number is positive, negative, or zero.
If the number is greater than 0
, the code inside the if
block is executed. If the number is less than 0
, the code inside the else if
block is executed. Otherwise, the code inside the else
block is executed.
Nested if
statement
A nested if
statement is an if
statement within another if
statement. It allows you to make more complex decisions based on multiple conditions.
Syntax
// outer if statement
if (condition1) {
// statements
// inner if statement
if (condition2) {
// statements
}
}
Notes:
- We can add
else
andelse if
statements to the innerif
statement as required. - The inner
if
statement can also be inserted inside the outerelse
orelse if
statements (if they exist). - We can nest multiple layers of
if
statements.
Program
// C++ program to find if an integer is positive, negative or zero // using nested if statements #include <iostream> using namespace std; int main() { int num; cout << "Enter an integer: "; cin >> num; // outer if condition if (num != 0) { // inner if condition if (num > 0) { cout << "The number is positive." << endl; } // inner else condition else { cout << "The number is negative." << endl; } } // outer else condition else { cout << "The number is 0 and it is neither positive nor negative." << endl; } cout << "This line is always printed." << endl; return 0; }
Output
Enter an integer: -35
The number is negative.
This line is always printed.
We hope this article helped you to understand about C++ if-else Statement in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.