Hi everyone, inside this article we will see about C++ Operators.
C++ operators are symbols that perform specific operations on variables and values in a program. They can be used to manipulate data, make decisions, and control the flow of a program.
There can be many types of operations like arithmetic, logical, bitwise etc.
Types of Operators
Here are some of the most common C++ operators:
Arithmetic operators: +, -, *, /, % (modulus), ++ (increment), — (decrement)
Relational operators: ==, !=, >, <, >=, <=
Logical operators: && (AND), || (OR), ! (NOT)
Assignment operators: =, +=, -=, *=, /=, %=, <<=, >>=, &=, ^=, |=
Bitwise operators: & (AND), | (OR), ^ (XOR), ~ (NOT), << (left shift), >> (right shift)
Conditional operator: ?:
sizeof operator
Pointer operators: * (dereference), & (address of)
Member access operators: . (dot operator), -> (arrow operator)
Scope resolution operator: ::
Note: The above list contains some of the most commonly used C++ operators. The C++ language has a wide range of operators, and the specific operators used in a program will depend on the requirements and goals of the code.
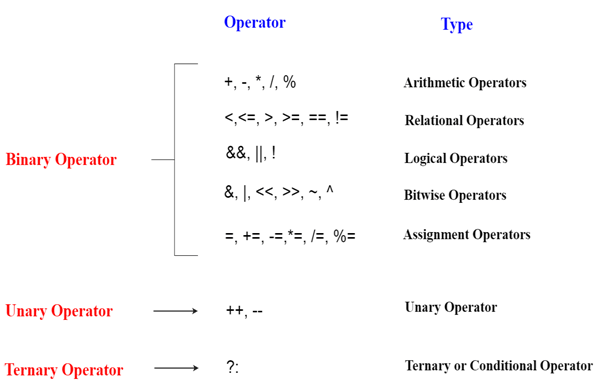
Precedence of Operators in C++
The precedence of operators in C++ determines the order in which operations are performed in a complex expression. If multiple operations are performed in a single expression, the operator with the highest precedence is evaluated first.
Here is the precedence order of operators in C++, from highest to lowest:
Postfix increment (++) and postfix decrement (–)
Unary plus (+) and unary minus (-)
Logical negation (!)
Bitwise negation (~)
Prefix increment (++) and prefix decrement (–)
Indirection (*) and address of (&)
Type cast (C-style type cast, C++-style type cast)
Multiplicative: *, /, %
Additive: +, –
Shift: <<, >>
Relational: <, <=, >, >=
Equality: ==, !=
Bitwise AND (&)
Bitwise XOR (^)
Bitwise OR (|)
Logical AND (&&)
Logical OR (||)
Ternary operator (? : )
Assignment: =, +=, -=, *=, /=, %=, &=, ^=, |=, <<=, >>=
Comma (,)
Note: Parentheses can be used to override the default operator precedence and explicitly specify the order in which operations are performed. Within a set of parentheses, operations are performed from left to right.
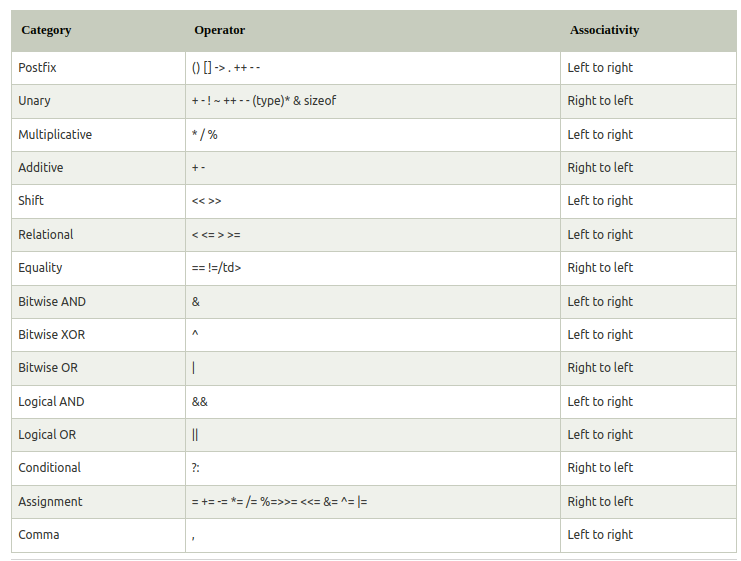
We hope this article helped you to understand about C++ Operators in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.