Hi everyone, inside this article we will see about C++ do-while loop Statement.
The do-while loop in C++ is similar to the while loop, with the difference being that the condition is evaluated after each iteration of the loop. This means that the body of the loop is guaranteed to be executed at least once, regardless of the condition.
Syntax & Explanation
It is defined using the following syntax:
do {
statement(s);
} while (condition);
statement(s)
: This is the body of the loop, executed repeatedly as long as the condition is true.
condition
: This is evaluated after each iteration of the loop. If the condition is true, the loop continues to execute. If it’s false, the loop stops executing.
Here, a pictorial representation of do-while
loop statement:
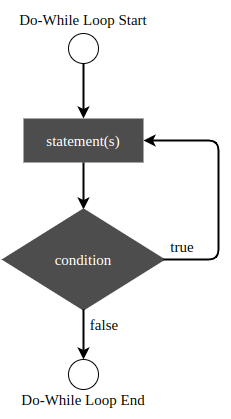
A Basic Program
Here’s a simple program in C++ that demonstrates the use of a do-while loop:
#include <iostream> using namespace std; int main() { int i = 1; do { cout << i << " "; i++; } while (i <= 10); cout << endl; return 0; }
The output of this program will be:
1 2 3 4 5 6 7 8 9 10
In this program, the do-while loop iterates 10 times (from 1 to 10), printing each number to the screen. The condition i <= 10
is evaluated after each iteration, and the loop continues as long as i
is less than or equal to 10. The value of i
is incremented by 1 in each iteration using the i++
statement.
It’s important to note that the body of the do-while loop is executed at least once, even if the condition is false. In this example, the condition i <= 10
is true, so the loop executes 10 times.
C++ Nested do-while Loop
A nested do-while loop in C++ is a do-while loop inside another do-while loop. The inner do-while loop is executed completely for each iteration of the outer do-while loop. This allows you to perform a task multiple times, nested inside another task that is also being repeated.
Here’s an example of a nested do-while loop in C++:
#include <iostream> using namespace std; int main() { int i = 1; do { int j = 1; do { cout << i * j << " "; j++; } while (j <= 3); cout << endl; i++; } while (i <= 3); return 0; }
The output of this program will be:
1 2 3
2 4 6
3 6 9
In this program, the outer do-while loop iterates three times (from 1 to 3) and the inner do-while loop iterates three times for each iteration of the outer loop. The product of i
and j
is printed to the screen, resulting in a 3×3 multiplication table. The inner loop continues as long as j
is less than or equal to 3 and the outer loop continues as long as i
is less than or equal to 3.
C++ Infinitive do-while Loop
An infinite do-while loop in C++ is a do-while loop that never terminates, it runs forever. It is created by having a condition in the do-while loop that is always true.
Syntax
do {
statement(s);
} while (true);
The body of the loop (statement(s)
) will be executed repeatedly until the program is stopped manually or a break statement is used to exit the loop.
It’s important to use infinite loops with caution, as they can lead to an unresponsive program if not used correctly. It’s always better to use a loop that has a well-defined ending condition.
Here is an example of an infinite do-while loop in C++:
#include <iostream> using namespace std; int main() { do{ cout << "Infinitive do-while Loop"; } while(true); return 0; }
The output of this program will be:
Infinitive do-while Loop
Infinitive do-while Loop
Infinitive do-while Loop
Infinitive do-while Loop
Infinitive do-while Loop
ctrl+c
We hope this article helped you to understand about C++ do-while loop in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.