Hi everyone, inside this article we will see about About C++ Pointers.
In C++, a pointer is a variable that stores the memory address of another variable.
Pointers are used to indirectly access the memory location of a variable and are an essential feature of C++ programming.
Syntax of Declaration
The pointer in C++ language can be declared using ∗ (asterisk symbol). The syntax of a pointer variable in C++ is as follows:
datatype *pointerName;
where datatype
is the data type of the variable that the pointer points to, and pointerName
is the name of the pointer variable.
For example, to declare a pointer variable that points to an integer, the syntax would be:
int *ptr;
This declares a pointer variable named ptr
that points to an integer.
To assign the address of a variable to a pointer variable, we use the address-of operator &
. For example, to assign the address of an integer variable x
to the pointer variable ptr
, we would use the following statement:
ptr = &x;
Symbols Used in C++ Pointer
Here are some common symbols used in C++ pointers along with their meanings:
Symbol | Meaning |
---|---|
* | Dereference operator (used to access the value pointed to by a pointer) |
& | Address-of operator (used to get the memory address of a variable) |
-> | Member access operator (used to access the members of an object pointed to by a pointer) |
++ | Increment operator (used to increment the memory address pointed to by a pointer) |
-- | Decrement operator (used to decrement the memory address pointed to by a pointer) |
[] | Array subscript operator (used to access the elements of an array pointed to by a pointer) |
sizeof | Returns the size (in bytes) of a data type or variable |
nullptr | A null pointer value |
Note that the meaning of these symbols may vary depending on the context in which they are used. For example, the *
symbol can be used both to declare a pointer variable and to dereference a pointer, and the &
symbol can be used both to take the address of a variable and to declare a reference variable.
How to Use C++ Pointer
In C++, pointers are used to indirectly access the memory location of a variable.
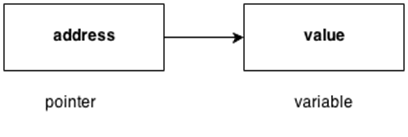
Here are some common use cases for pointers in C++:
Dynamic memory allocation: Pointers are commonly used to dynamically allocate memory at runtime. This allows for the creation of data structures that can grow or shrink in size as needed.
Pass-by-reference: Pointers can be used to pass values to functions by reference, allowing the function to modify the value of the variable passed in.
Pointer arithmetic: Pointers can be used to perform arithmetic operations on memory addresses, allowing for the efficient traversal of data structures such as arrays and linked lists.
Here is an example program that demonstrates how to use pointers in C++:
#include <iostream> using namespace std; int main() { int x = 10; // Declare and initialize a variable int *ptr; // Declare a pointer variable ptr = &x; // Assign the address of x to the pointer variable cout << "The value of x is: " << x << endl; cout << "The memory address of x is: " << &x << endl; cout << "The value of ptr is: " << ptr << endl; cout << "The value of *ptr is: " << *ptr << endl; *ptr = 20; // Change the value of x using the pointer variable cout << "The value of x is now: " << x << endl; return 0; }
In this program, we declare a variable x
and initialize it to 10. We then declare a pointer variable ptr
and assign the memory address of x
to it using the address-of operator &
. We print the value of x
, the memory address of x
, the value of ptr
, and the value pointed to by ptr
using the dereference operator *
.
We then change the value of x
to 20 using the pointer variable ptr
and print the new value of x
.
The output of this program will be:
The value of x is: 10
The memory address of x is: 0x7ffee5795b5c
The value of ptr is: 0x7ffee5795b5c
The value of *ptr is: 10
The value of x is now: 20
In this example, we can see how to use a pointer variable to indirectly access the value of a variable, and how to modify the value of a variable using a pointer.
We hope this article helped you to understand about About C++ Pointers in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.