Hi everyone, inside this article we will see the concept about Queue Data Structure.
In computer science, a queue is a linear data structure that follows the First-In-First-Out (FIFO) principle. In other words, the first element that is inserted into the queue will be the first element to be removed. A queue can be thought of as a collection of elements in which the elements are added at one end, and removed from the other end.
Queues are used in a wide range of computer science applications such as breadth-first search algorithms, task scheduling, message queues, and many more.
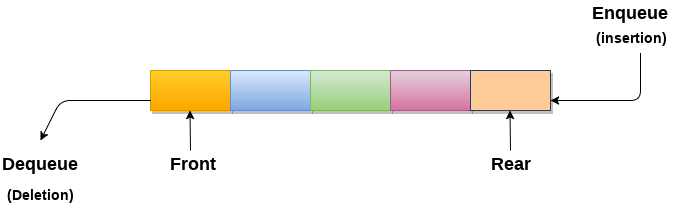
A queue has two primary operations, which are:
- Enqueue: This operation adds an element to the back of the queue.
- Dequeue: This operation removes the element from the front of the queue.
In addition to these two primary operations, there are a few more operations that can be performed on a queue, such as:
- Peek: This operation returns the front element of the queue without removing it.
- Size: This operation returns the number of elements in the queue.
- Is Empty: This operation checks whether the queue is empty or not.
Queues can be implemented using arrays or linked lists. An array-based queue has a fixed size, whereas a linked list-based queue can grow and shrink dynamically as elements are added or removed. Choosing the appropriate implementation depends on the requirements of the specific application.
Key points about Queues in Data Structure
Here are some key points related to queues in data structure:
- A queue is a linear data structure that follows the First-In-First-Out (FIFO) principle.
- A queue has two primary operations: enqueue and dequeue. The enqueue operation adds an element to the back of the queue, and the dequeue operation removes the front element from the queue.
- In addition to enqueue and dequeue, there are other operations that can be performed on a queue, such as peek, size, and is empty.
- Queues are used in a wide range of computer science applications such as breadth-first search algorithms, task scheduling, message queues, and many more.
- Queues can be implemented using arrays or linked lists. An array-based queue has a fixed size, whereas a linked list-based queue can grow and shrink dynamically as elements are added or removed.
- Queues are often used in conjunction with other data structures, such as stacks and trees, to solve complex problems.
- The time complexity of the enqueue, dequeue, peek, size, and is empty operations in a queue is O(1) in the average and worst case.
- A circular queue is a variation of a queue that allows the elements to wrap around from the end to the beginning, which can be useful in some applications.
- Double-ended queues (dequeues) are a variation of queues that allow elements to be added or removed from both ends of the queue.
- Priority queues are a variation of queues that prioritize elements based on a specific order, such as their value or priority level.
Types of Queues in Data Structure
There are several types of queues in data structure, some of the most common types include:
- Simple Queue: A simple queue is a basic queue that follows the First-In-First-Out (FIFO) principle. It allows elements to be added to the rear end and removed from the front end.
- Circular Queue: A circular queue is a variation of a simple queue where the rear and front ends are connected to form a circle. This allows elements to be added or removed from both ends, and it is useful when there is a fixed size for the queue.
- Priority Queue: A priority queue is a type of queue where each element is associated with a priority. Elements with higher priority are dequeued first. Priority queues are often used in algorithms like Dijkstra’s shortest path algorithm and Huffman coding.
- Double-Ended Queue (Deque): A deque is a type of queue that allows insertion and deletion of elements from both front and rear ends. This allows for more flexibility in data manipulation, and it is used in applications such as simulations and process scheduling.
- De-prioritized Queue: A de-prioritized queue is a type of priority queue where the priorities of elements change dynamically. The priorities of the elements in the queue are adjusted based on certain conditions.
- Delay Queue: A delay queue is a type of queue that holds elements for a specified amount of time before releasing them. Delay queues are often used in applications like message queuing and scheduling.
- Concurrent Queue: A concurrent queue is a type of queue that allows multiple threads to access the queue simultaneously without causing data corruption or other synchronization issues.
These are some of the common types of queues in data structure, each with its own unique characteristics and applications.
We hope this article helped you to understand about Queue Data Structure in a very detailed way.
Online Web Tutor invites you to try Skillshike! Learn CakePHP, Laravel, CodeIgniter, Node Js, MySQL, Authentication, RESTful Web Services, etc into a depth level. Master the Coding Skills to Become an Expert in PHP Web Development. So, Search your favourite course and enroll now.
If you liked this article, then please subscribe to our YouTube Channel for PHP & it’s framework, WordPress, Node Js video tutorials. You can also find us on Twitter and Facebook.